Program Raspberry Pi I/O as with 8052
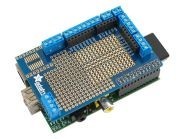
The problem:Everybody that has been programming with 8052 processors family has lots of code that would like to reuse with modern microprocessors and/or compilers.The problem comes when porting the old-fashion I/O pins calling, to newer functions.Traditionally, to access to pin D3 of Port 0 we must first define:
The problem:
Everybody that has been programming with 8052 processors family has lots of code that would like to reuse with modern microprocessors and/or compilers.
The problem comes when porting the old-fashion I/O pins calling, to newer functions.
Traditionally, to access to pin D3 of Port 0 we must first define:
sbit SPI_CLK = P0^3; (Keil compler)
or
#define SPI_CLK P0_3 (SDCC, Small Device C Compiler)
Afterwards, to assign a value to the pin or to read a value from de pin, we can simply write:
SPI_CLK = Value;
Value = SPI_CLK;
Nowadays, if you try to manage I/O pins with a Raspberry Pi, you can use some libraries like bcm2835 or wiringpi, but pin calling is completely different.
Focusing on bcm2835 libraries, to write a pin you must program:
bcm2835_gpio_write(SPI_CLK, 1);
and to read a pin,
Value = bcm2835_gpio_lev(SPI_CLK);
Then, porting from
PIN1 = PIN2 | PIN3;
to
bcm2835_gpio_write(PIN1, bcm2835_gpio_lev(PIN2) | bcm2835_gpio_lev(PIN3) );
can convert a good code to an unreadable one, not to mention that the probability of making an error in the conversion process approaches 1.
I found a solution making a simple C++ class library that allows us to reuse the code as it was written, with minor modifications.
Usage:
See the attached table where you can compare the tree methods: past, present and future.
Test:
There is attached too, a sample program to test inputs and outputs.
It blinks a LED connected to the pins GPIO17 (pin 11 of connector P1) and GND (pin 9).
Connecting GPIO18 (pin 12 of connector P1) to GND, the LED blinks faster.
Uncompress the contents of cio_sample.tar file in any Raspberry Pi folder. Then
pi@raspberrypi ~/myfolder $ make
pi@raspberrypi ~/myfolder $ sudo ./cio_sample
Don’t forget to write sudo, otherwise you don’t have permission to access the address of I/O ports.
Links:
http://www.keil.com/c51
http://sdcc.sourceforge.net
http://www.airspayce.com/mikem/bcm2835/index.html
https://projects.drogon.net/raspberry-pi/wiringpi/
Diskussion (0 Kommentare)