AVR Software Defined Radio (2) - Sampling Signals
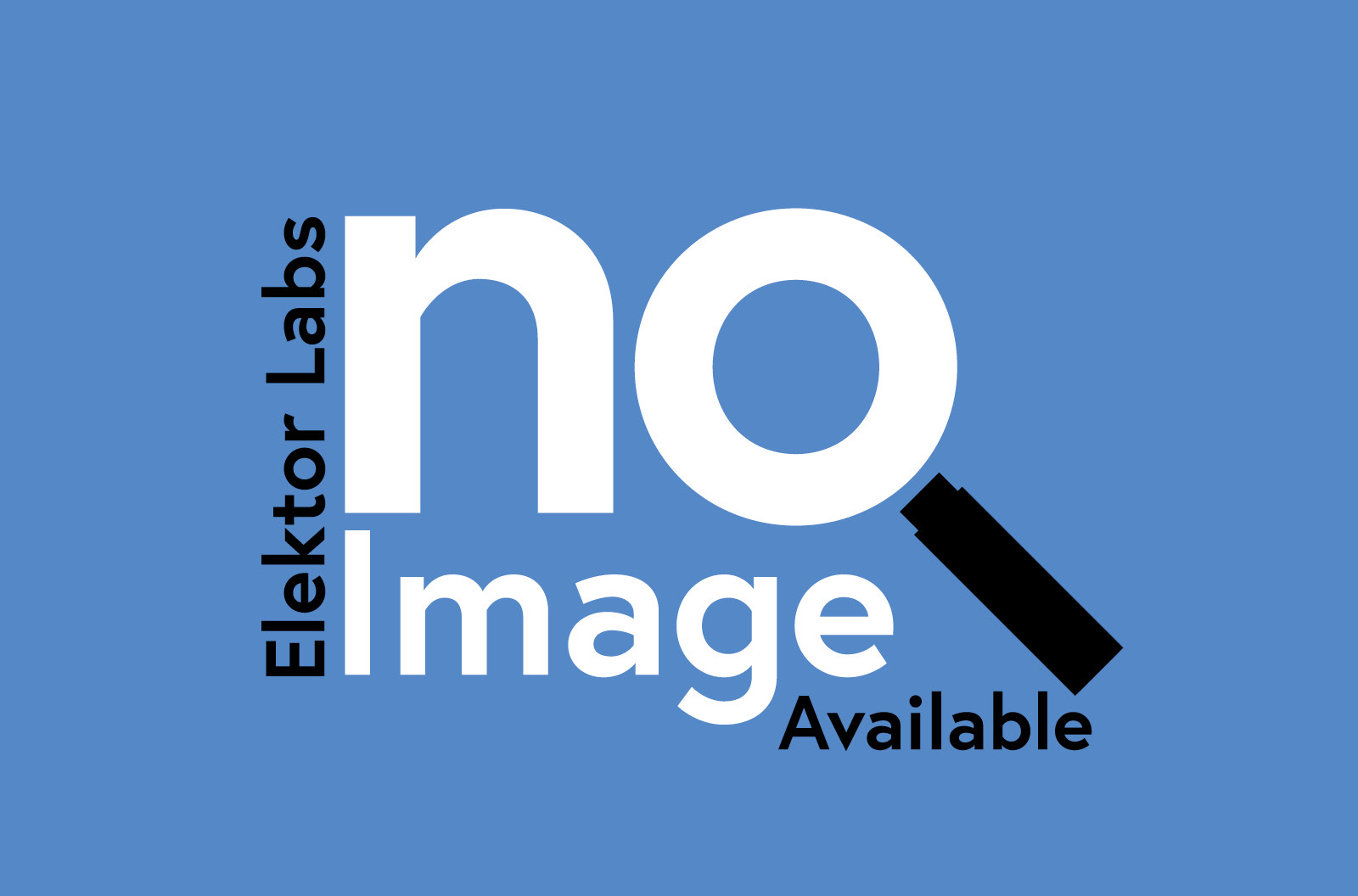
As this series shows, the popular AVR microcontroller can be used for digital signal processing tasks. Here we will use an ATmega88 to sample amplitude- and phase-modulated signals which we can either synthesise ourselves or fish out of the ether. We can even operate at frequencies of above 100 kHz. How does it work?
As this series shows, the popular AVR microcontroller can be used for digital signal processing tasks. Here we will use an ATmega88 to sample amplitude- and phase-modulated signals which we can either synthesise ourselves or fish out of the ether. We can even operate at frequencies of above 100 kHz. How does it work? Read on to find out, in theory and in practice.
By Martin Ossmann
A carrier wave can be used to send audio or data through the ether by modulating it in amplitude, frequency or phase. In a ‘software defined radio’ the first thing that happens is that the received signal is sampled; then a processor carries out the necessary calculations to recover the modulating signal. In the case of data transmission, we then decode the signal into a stream of bits. To understand how this all works, we will first look at how an analogue receiver operates.
Reception, the analogue way
The input stage of many modern receivers looks like Figure 1 (where we have not shown the first stage of ‘preselection’ filtering). It works as follows: suppose first that we want to receive a signal at Uin with a frequency of fRX = 2 kHz. We set the frequency fLO of the local oscillator (or ‘LO’) also to fLO = 2 kHz. In the upper branch of the circuit (which is called the ‘in-phase’ or ‘I’ channel) the input signal is multiplicatively mixed with the cosine wave produced by the LO. This produces a DC component X, which passes unchanged through the low-pass filter that follows. A component at 4 kHz is also produced, which is removed by the low-pass filter. The value of X depends on the amplitude A and phase φ of the input signal, the phase being measured relative to the output of the local oscillator. More precisely, ignoring any gain in the low-pass filter, we have X = A cos φ. If the input signal is exactly in phase with the cosine output of the local oscillator, X is maximised: this is why this branch is labelled ‘in-phase’.
Much the same happens in the lower branch of the diagram. The difference is that the input is mixed with a sine signal (the cosine signal with a 90 ° phase shift). The value of Y depends again on the amplitude A and phase φ of the input signal, and we have Y = A sin φ. Y is maximised when the input signal is 90 ° phase shifted with respect to the cosine output of the LO. For this reason, the lower branch is called the ‘quadrature’ channel.
Figure 2 shows the relationships graphically. The receiver can calculate the amplitude A and phase φ of the input signal from the values of X and Y.
Let’s get digital
Now let’s consider what happens when all the signals involved are sampled at a sample rate fS = 8 kHz, exactly four times the frequency of the input signal (see Figure 3).
The process of sampling converts the continuous-time input signal into a sequence of numbers. If the input signal Uin is a cosine wave with amplitude A and frequency 2 kHz (at the top of Figure 3), sampling generates the sequence of values Uin = A, 0, –A, 0, A and so on. The values repeat every four samples, because we are sampling at four times the input frequency.
Let us look first at the in-phase channel and sample the cosine output signal of the local oscillator. The sequence of samples is LOCOS = 1, 0, –1, 0, 1, .... Again, this repeats every four samples. The mixer multiplies the samples of Uin by those of LOCOS. The result is the sequence U = A, 0, A, 0, A, .... After the mixer this sequence is passed through a low-pass filter. We can construct a simple low-pass filter by calculating a rolling average of sequences of four consecutive samples of U. For simplicity we multiply this result by 2, and the output of the filter is then X = A, A, A, A, A, ...; in other words, the output is a constant with value A. X can be thought of as samples of a DC level of A, where A is exactly the amplitude of the original input signal.
Now we turn to the quadrature branch. The inputs to this branch’s mixer are the sequences Uin = A, 0, –A, 0, A, ... and LOSIN = 0, 1, 0, –1, 0, .... The product of these sequences is V = 0, 0, 0, 0, 0, ...; in other words, the constant value zero. The result of low-pass filtering V will also be zero.
The same argument can be applied when the input signal is a sine wave Uin = A sin (2π · 2 kHz · t), giving the results X = 0 and Y = A. This shows that our discrete-time I-Q mixer works in just the same way as the classical analogue I-Q mixer described above. We have also seen that if the sample rate is four times the signal frequency, the output signals of the LO only take on the values zero, plus one and minus one. This in turn means that the digital mixer does not need a multiplier: we simply need to add and subtract the relevant input samples in the low-pass filter to calculate the values of X and Y.
The hardware...
A simple front end circuit (Figure 4) was designed to test this idea on an AVR microcontroller. The analogue-to-digital converter inside the ATmega88 is used to sample the input signal Uin and digitise it. The firmware then carries out the necessary calculations and the results, X and Y, are output using PWM on pins OC0A and OC0B. To attenuate the PWM frequency component in these output signals, each is passed though a two-stage RC low-pass filter.
The circuit is straightforward enough to be built on a small piece of prototyping board. Things are made even easier if the Elektor universal receiver board is used: its circuit diagram is shown in Figure 5. As was the case for the signal generator described in the first part of this series, this is available as a kit including the printed circuit board (Figure 6) and all components. This is a good option, as populating the board is not a tricky task. As you can see from the circuit diagram the universal receiver board includes all the components of the simple front end, but also allows for a wide range of additional future possibilities that we will look at later on in this series. For example, it is possible to connect an active ferrite antenna: an example of such an antenna is again available as an Elektor kit, and the electronics and printed circuit board will be described in the next instalment in this series.
... and the software...
The software, as always, is available as source code and as a hex file for download at the end of this article. For our first experiment the software we use is called EXP-SimpleFrontend-2kHz-IQout-V01.c.
The program samples the input signal at a rate of 20 MHz / 5000 = 8 kHz. The signal is then mixed with a 2 kHz signal. A simple low-pass filter then produces the X and Y outputs, which we can also label as the quadrature components I and Q.
Listing 1 shows the heart of this routine. The timer variable sampleTimealways counts cyclically from 0 to 3, and thus represents the current phase of the local oscillator. The variables Uand Vare used to hold the values that are obtained by multiplying the input value ADCvby the cosine sequence [1, 0, –1, 0] and the sine sequence [0, 1, 0, –1] respectively. The values of Uand Vare then fed into a simple low-pass filter that calculates a rolling average over sets of four samples. The results of this calculation, Xand Y, are the in-phase and quadrature components of the signal and are written to the PWM registers OCR0A and OCR0B respectively.
Listing 1: Calculating the quadrature components
U=0 ;
if (sampleTime==0){ U= ADCv ; }
if (sampleTime==2){ U= - ADCv ; }
U3=U2 ; U2=U1 ; U1=U0 ; U0=U ;
X=U0+U1+U2+U3 ;
OCR0A=128+X/8 ;
V=0 ;
if (sampleTime==1){ V= ADCv ; }
if (sampleTime==3){ V= - ADCv ; }
V3=V2 ; V2=V1 ; V1=V0 ; V0=V ;
Y=V0+V1+V2+V3 ;
OCR0B=128+Y/8 ;
... and, finally, testing
To test the receiver, we feed a sinewave signal from our signal generator into the front end hardware via a 10 kΩ potentiometer to allow adjustable attenuation. We use the SINE OUT (K3) output of the signal generator and the program called EXP-SinusGenerator-DDS-ASM-C-V01; the wiper of the potentiometer is connected to input ADC0 on the receiver board.
The analogue outputs DAC1 and DAC2 are connected to an oscilloscope operating in X-Y mode. Then we instruct the signal generator over its RS-232 interface to generate a 2 kHz sinewave and adjust the amplitude of the signal using the potentiometer until the red LED (D3 in Figure 5) does not quite light. The front end is then being driven to its maximum level, just short of clipping. The oscilloscope should show a single point that moves slowly in a circle. In theory the point should be stationary, but because the oscillator controlling the front end is not running at exactly the same frequency as that controlling the signal generator, the point will move.
To see the effect more clearly, adjust the signal generator to produce a frequency of 2005 Hz. Then the point will move in a circle making five revolutions per second. With the signal generator set to 1995 Hz, the point will again move at five revolutions per second, but in the opposite direction. Adjusting the amplitude of the input signal using the potentiometer affects the radius of the circle in which the point moves.
Our ‘I-Q demodulator’ has mixed the signal in the band around 2 kHz down to a centre frequency of 0 Hz. Signals in sidebands above and below 2 kHz are now distinguished in the direction of rotation of the point on the oscilloscope display. Now frequencies around 2 kHz are of relatively little practical interest: more interesting are frequencies in the longwave bands used for sending data by various transmitters. This requires one further small step, as we shall see in the next section.
The road to RF
The Nyquist-Shannon sampling theorem states that a sample rate of at least 2 f is required to represent losslessly a signal containing frequency components up to a frequency f. Using a lower sample rate than this is called ‘undersampling’ or ‘sub-Nyquist sampling’.
Take a look at Figure 7. The time interval illustrated is 1 ms long. The upper black curve is a cosine wave, with the corresponding sine wave below, both at 10 kHz. As before, the signal is sampled at 8 kSa/s (kilosamples per second), giving sample values indicated by the small blue circles. With conventional sampling we need at least two samples per period of the 10 kHz signal, but here we have less than one sample per period: hence the signal is undersampled. The sequence of sample values that we obtain is [1, 0, –1, 0, 1, 0, –1, 0, 1] for the upper signal and [0, 1, 0, –1, 0, 1, 0, –1, 0] for the lower one. Superimposed on the figure, in red, are 2 kHz cosine- and sinewaves. For these signals the sample rate of 8 kSa/s satisfies the sampling theorem; but the surprise is that the 2 kHz signal and the 10 kHz signal give rise to the same set of sample values. This means that a 10 kHz signal sampled at 8 kSa/s is indistinguishable from a 2 kHz signal sampled at the same rate. In turn this means that our front end, which uses an 8 kSa/s sample clock, can equally well be used to demodulate signals at 10 kHz.
There is of course a full theoretical analysis of the above phenomenon, known as the Nyquist-Shannon sampling theorem for bandpass signals. One consequence of this theorem is that a sampled bandpass signal with bandwidth B starting at a multiple of the sample rate fS can be reconstructed perfectly as long as B < fS / 2. In particular, we can reconstruct a bandpass signal with components stretching from n × fS to n × fS + fS / 2 for any chosen integer n; or, stated another way, a bandpass signal centred at n × fS + fS / 4 with total bandwidth up to fS / 2. In particular, if we have a sample rate of 8 kHz we can demodulate signals equally well around any of the following frequencies: 2 kHz, 2 kHz + 1 × 8 kHz = 10 kHz, 2 kHz + 2 × 8 kHz = 18 kHz, 2 kHz + 3 × 8 kHz = 26 kHz, ..., 2 kHz + 20 × 8 kHz = 162 kHz, and so on. We can easily test this, for example by setting the signal generator frequency to 26005 Hz.
For undersampling like this to be successful the signal being sampled must be band-limited, for example by the insertion of a bandpass filter in front of the AVR. Image frequencies at n × fS – fS / 4 are also received: in our case these images are at 8 kHz – 2 kHz = 6 kHz, 2 × 8 kHz – 2 kHz = 14 kHz, ..., 25 × 8 kHz – 2 kHz = 198 kHz, and so on.
So far we have assumed that the signal is perfectly digitised, and of course that is not the case. The sample-and-hold stage in any ADC has a so-called ‘aperture’, and a result of this is that it is not possible to mix down very high frequencies using the AVR. However, in the next part of this series, we will see how a signal transmitted by the BBC on 648 kHz (in the mediumwave band!) can easily be decoded.
Amplitude and phase
The signals X and Y give the strength of the in-phase and quadrature components of the input signal. However, we are rather more interested in its amplitude A and phase φ, since one of our ultimate aims is to decode amplitude- and phase-modulated signals. If we had fast floating-point arithmetic we could compute the amplitude and phase from the X and Y coordinates using the following two statements.
A = sqrt(X * X + Y * Y);
PHI = atan2(Y, X);
The program EXP-SimpleFrontend-2kHz-Phase-Ampl-V01.ccalculates phase and amplitude using a rather more efficient method and outputs the results on DAC1 and DAC2. The output voltage level representing amplitude is logarithmically scaled, enabling a direct conversion to dB. Figure 8 shows how the phase of the signal transmitted by the BBC on 198 kHz changes over time. The signal was received using an active ferrite antenna whose amplified output is fed into the receiver’s front end. Antenna input ANT2 on the receiver board can be used for this purpose, with pin 1 of K4 linked to pin 2 of K5 so that the signal appears on input ADC0 of the microcontroller. Again we use a sample rate of 8 kHz. Since 198 kHz = 25 × 8 kHz – 2 kHz, the signal of interest is mixed down to 2 kHz. The BBC transmission includes digital data sent using a phase modulation of ±22.5 degrees at a rate of 25 bit/s. This digital modulation can clearly be observed in the mixed-down signal.
FM, PM and AM — with PWM
Next we want to try to generate some modulated signals ourselves. First install the program EXP-SQTX-125kHz-PWMa-V01.cinto the signal generator’s microcontroller. The 125 kHz squarewave output by this code is filtered using the resonant circuit described in part 1 of this series into a sinewave. Feed this signal into the ADC0 input of the receiver front end. This circuit arrangement will be used for the following experiments.
With the program EXP-SimpleFrontend-125kHz-Phase-Ampl-V01.crunning in the front end the outputs will represent the amplitude and phase of the received 125 kHz signal. Again the amplitude output is logarithmically scaled so that a wider dynamic range can be represented: an output voltage of 4 V corresponds to an input amplitude of 1 VPP and a step of 20 dB in amplitude gives a change of 1 V in the output level. A phase output voltage of 5 V represents a phase angle of exactly 360 °.
In the PWM code mentioned above a range of different modulation schemes and data sequences can be selected using #define preprocessor directives. First we will try straightforward PWM, where the frequency of a squarewave remains constant but its mark-space ratio is modulated. The bit transmission routine simply loads the modulating value into PWM register OCR1A (Listing 2).
Listing 2: Modulation using simple PWM
void bitSend(uint8_t theBit){
if (theBit) {
OCR1A = 80 ;
}
else {
OCR1A = 20 ;
}
}
The period is fixed at 160, which means that the output frequency is 20 MHz / 160 = 125 kHz. The duty cycle is switched between D = 80 / 160 = 0.5 and D = 20 / 160 = 0.125. Figure 9 is an oscilloscope trace showing the resulting variation in amplitude and phase. It is clear that both of these quantities are modulated, the amplitude varying by 0.4 V (which, as 1 V corresponds to 20 dB, means 8 dB). The phase variation is trickier to analyse. The first point to note is that the phase jumps, in line with the data bit being transmitted, by about 0.92 V. This corresponds to 0.92 / 5 × 360 = 66 degrees. On top of this is superimposed a slow sawtooth signal with a slope (read from the oscilloscope trace) of about 5 V in 0.5 s. Now, 5 V corresponds to a phase angle of 360 degrees, and so the phase angle is making approximately two complete revolutions every second. The reason for this is again that the transmitter and receiver have very slightly different ideas of what ‘125 kHz’ means: in fact, in this case they differ by 2 Hz. We will need to be careful to allow for this effect in future experiments. An error of 2 Hz in 125 kHz corresponds to 16 ppm, which is rather better than the typical ±50 ppm tolerance of a crystal oscillator. It is possible to compensate for this drift using a PLL control loop to drive the oscillator in the receiver.
A more refined kind of modulation
The above is all very well, but we would naturally like to implement pure amplitude modulation. And likewise, when implementing phase modulation, we would like to alter only the phase of the signal and not the amplitude. To that end we need to understand better what is happening in the PWM system.
Figure 10 shows the squarewave signal with the two different mark-space ratios D = 0.5 and D = 0.125, in each case accompanied by the sinewave signal that results after extracting just the fundamental component using the 125 kHz resonant circuit. The filtered sinewave reaches its peak exactly in the middle of the squarewave pulse. The mid-point moves when the mark-space ratio is changed, and so there is a phase shift when the mark-space ratio is changed.
In our example, when we use a mark-space ratio of D = 0.5 the phase angle is 0.5 × 180 degrees, or 90 degrees. When using a mark-space ratio D = 0.125 the corresponding phase angle is 0.125 × 180 degrees, or 22.5 degrees. The difference between these two phase angles is 90 – 22.5 = 67.5 degrees, which is in close agreement with the measured value from Figure 9 of 66 degrees.
With a little more mathematics we can also calculate the amplitude variation as a function of the mark-space ratio D. The peak-to-peak amplitude  of the sinewave is given by the formula
 = 5 V × (4 / π) sin πD
and from this we can determine that the amplitude ratio between the cases D = 0.5 and D = 0.125 should be 0.3826834... = –8.343 dB. Again, this is in good agreement with the value of 8 dB measured from Figure 9.
The relationship given above can also be illustrated graphically. Figure 11 shows how we can determine the amplitude that we will obtain with a given mark-space ratio D. Construct a line from the origin of the plot inclined at an angle of D × 180 degrees to the point where it intersects the indicated circle. The length of this line then gives the amplitude. As can be seen, the amplitude is maximal when D = 0.5, and for any R, mark-space ratios of D = 0.5 – R, and D = 0.5 + R give the same amplitudes. We can therefore use a pair of such values to create pure phase modulation without an amplitude component, and this is exactly what our PWM generator does when it is switched to PM mode using a #define directive. The essential part of this code is shown in Listing 3.
Listing 3: PWM modified for pure phase modulation
void bitSend(uint8_t theBit){
if (theBit) {
OCR1A = 80+10 ;
}
else {
OCR1A = 80-10 ;
}
}
Figure 12 shows the result. The phase switches constantly between the two values that represent zero and one. Superimposed on this is a small drift due to the frequency error. The amplitude (yellow curve) is constant.
In the next part of this series we will look at how we can achieve pure amplitude modulation. However, we will not just be looking at this in theory: we will also implement a DCF test transmitter and receive DCF signals. DCF is a German time standard transmitter.
Diskussion (2 Kommentare)