Entwickeln Sie Ihre eigenen Bluetooth Low Energy Anwendungen (Buch in englischer Sprache)
über
Bluetooth Low Energy (BLE) Funkchips sind vom Raspberry Pi bis zur Glühbirne überall anzutreffen. BLE ist eine komplizierte Technologie mit einer umfassenden Spezifikation. Glücklicherweise sind die Grundlagen recht leicht zugänglich. Der progressive und systematische Ansatz in diesem neuen Buch von Elektor wird Sie bei der Beherrschung dieser drahtlosen Kommunikationstechnik, die für die Arbeit in Low-Power-Szenarien unerlässlich ist, weit bringen.
Weiter auf Englisch: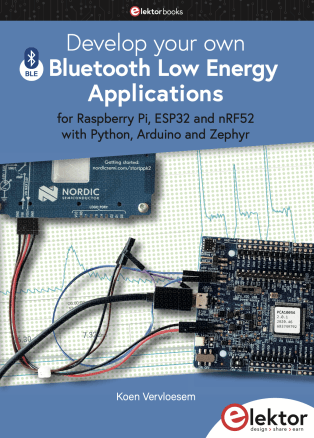
for Raspberry Pi, ESP32 and nRF52
Various low-power scenarios Bluetooth Low Energy (BLE) radio chips are ubiquitous, from Raspberry Pi down to light bulbs. BLE is an elaborate technology with a comprehensive specification. Fortunately, the basics are quite accessible.
The progressive and systematic approach in this new book from Elektor will get you far in mastering this wireless communication technique, which is essential for working in low-power scenarios.A New Book with Hardware and Software Complements!
In this book by Elektor author Koen Vervloesem, you’ll learn how to:- Discover BLE devices in the neighborhood by listening to their "advertisements".
- Create your own BLE devices advertising data.
- Connect to BLE devices such as heart rate monitors and proximity reporters.
- Create secure connections to BLE devices with encryption and authentication.
- Understand BLE service and profile specifications and implement them.
- Reverse engineer a BLE device with a proprietary implementation and control it with your own software.
- Make your BLE devices use as little power as possible.
- On a Raspberry Pi or PC, you do it with Python and the Bleak library.
- On the ESP32 development boards from Espressif, you may use C++ and NimBLE-Arduino.
- On one of the development boards supported by the Zephyr real-time operating system, such as the nRF52 boards from Nordic Semiconductor, you'd use C.
Starting with a very little amount of theory, you’ll develop code right from the beginning. After you’ve completed this book, you’ll know enough to create your own BLE applications.
Example Program Listing from the Book
Develop your own BLE applications for Raspberry Pi, ESP32 and nRF52
(Note: in the book, the syntax of all listings is highlighted in color)
import asyncio
from uuid import UUID
from construct import Array, Byte, Const, Int8sl, Int16ub, Struct
from construct.core import ConstError
from bleak import BleakScanner
from bleak.backends.device import BLEDevice
from bleak.backends.scanner import AdvertisementData
ibeacon_format = Struct(
"type_length" / Const(b"\x02\x15"),
"uuid" / Array(16, Byte),
"major" / Int16ub,
"minor" / Int16ub,
"power" / Int8sl,
)
def device_found(
device: BLEDevice, advertisement_data: AdvertisementData
):
"""Decode iBeacon."""
try:
apple_data = advertisement_data.manufacturer_data[0x004C]
ibeacon = ibeacon_format.parse(apple_data)
uuid = UUID(bytes=bytes(ibeacon.uuid))
print(f"UUID : {uuid}")
print(f"Major : {ibeacon.major}")
print(f"Minor : {ibeacon.minor}")
print(f"TX power : {ibeacon.power} dBm")
print(f"RSSI : {device.rssi} dBm")
print(47 * "-")
except KeyError:
# Apple company ID (0x004c) not found
pass
except ConstError:
# No iBeacon (type 0x02 and length 0x15)
pass
async def main():
"""Scan for devices."""
scanner = BleakScanner()
scanner.register_detection_callback(device_found)
while True:
await scanner.start()
await asyncio.sleep(1.0)
await scanner.stop()
asyncio.run(main())
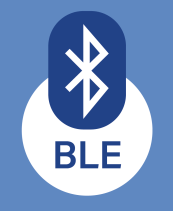
Concise Table of Contents
Chapter 1 • IntroductionLayered architecture
How to communicate with BLE devices
Advantages of BLE
Disadvantages of BLE
Platforms used in this book
The Arduino platform with NimBLE-Arduino for the ESP32
The Zephyr development environment for nRF5 devices
The nRF Connect for Desktop application
The nRF Connect mobile app
The Bluetooth Low Energy app in nRF Connect for Desktop
Wireshark and a BLE sniffer dongle
Advertising packets
Discovering advertisements with Bleak
Public and random Bluetooth addresses
The iBeacon specification
Decoding iBeacon advertisements using Bleak
Discovering advertisements with NimBLE-Arduino
Decoding manufacturer-specific data using NimBLE-Arduino
Broadcasting iBeacon advertisements with Zephyr
Broadcasting sensor data as manufacturer-specific data with Zephyr
Advertise scan response data with Zephyr
Attributes
Services, characteristics, and descriptors
Discovering services and characteristics with nRF Connect
A minimal GATT server
Discovering services and characteristics with Bleak
Reading and writing characteristics using Bleak
Notifications and indications
Creating a heart rate monitor with NimBLE-Arduino
Creating a GATT server with Zephyr
Receiving service data without a connection
Pairing and bonding
Security modes and levels
Encrypting the BLE connection to a Zephyr sensor
Authenticating a BLE connection
Privacy
Understanding a profile specification
Understanding a service specification
Understanding the definition of a characteristic
Implementing a Proximity Reporter in Zephyr
Implementing a Proximity Monitor in NimBLE-Arduino
Decompiling the mobile app
Sniffing BLE traffic between the LED badge and the mobile app
Writing arbitrary images to the LED badge using Bleak
Measuring an iBeacon’s power consumption
Lowering power consumption by disabling hardware
Lowering the power consumption by using a larger advertising interval
Estimating battery life
More about Bluetooth Low Energy
Some ideas for further exploration
16-bit UUID ranges
Verifying a product’s Bluetooth qualifications
Establishing a serial connection to a device over USB
Sniffing BLE traffic on your Android device using the Bluetooth HCI snoop log
Tips for specific hardware
Diskussion (0 Kommentare)